Hello, modules!
Module is a very exciting part of C++20. Similar to Go modules, C++20 modules can save the compiler from repeatedly scanning header files. This will make C++ code build faster and become more modular (independent of #include order). And the Hello World program becomes easier to write XD
This program is compiled using cl.exe 19.33 (MSVC v143, Visual Studio 2022). Gcc and Clang still don’t support importing standard library yet, and MSVC support is still experimental. But the future of C++ looks great!
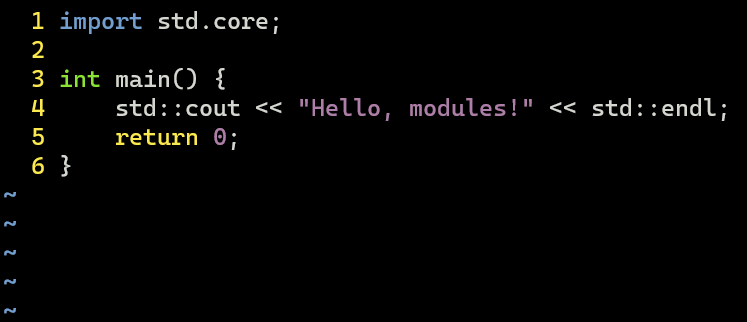
I learned C++98 in around 2014 (due to outdated C++ compilers in National Olympiad of Informatics: we were still using Ubuntu 10.10 (a non-LTS version, EOL in 2011) in competitions then because it still works). Since C++98, C++ has evolved significantly and helps programmers write modern, efficient code. The largest evolution is probably C++11 and C++20. Some impressive new features are:
auto
- Automatic type deduction, which saves us from typing something likestd::vector<std::vector<int> >::const_iterator
manually.constexpr
andconsteval
- Compile time evaluation. As C++ users, we want extreme performance!enum class
andstd::variant
- More structured enums and unions. Finally they are not just integer values.- Range-based for - I guess it’s syntactic sugar, but it can be life-saving!
- Smart pointers - RAII is the right way to handle memory (as well as other resources) in an efficient way.
- concepts - Makes generic programming more fun and reliable.
T&&
- RValue reference. Together with copy elision, we can make data flow more efficiently by removing redundant copy operations. (Which type isauto&&
?)- Lambda function
[] () {}
- Sounds useful with STL algorithms. - Ranges - More powerful “iterators”!
- Lots of cool stuff in STL (
std::thread
,std::atomic
,std::bind
,std::chrono
, and many others).